A good way to improve the overall user experience and performance of your website is to make your web-pages load faster. If you want to achieve this, you should be able to:
How Can I Improve Page Loading? Here Are Some Tips...
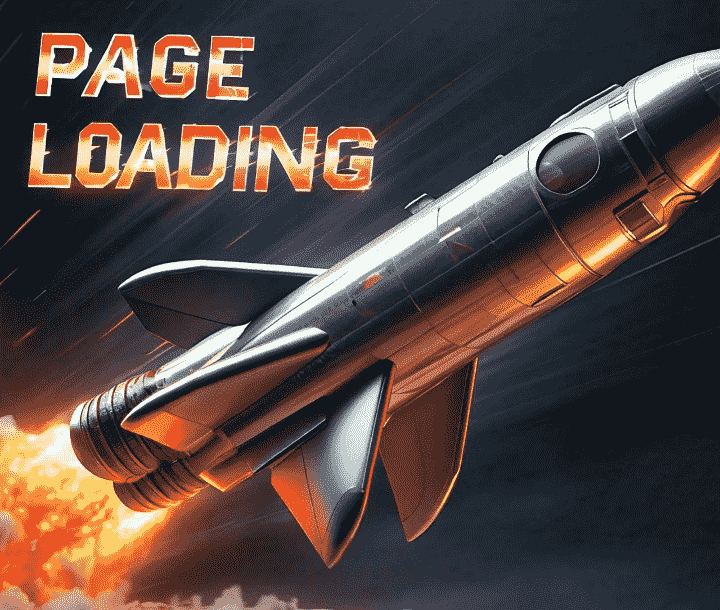
1. Optimize your images
Images are mostly large in size (sometimes >300kb), and if not optimized, it could significantly slow down load time.
How to Optimize?
-
Compress the images
:
There are a bunch of online tools that can be used to compress images to smaller sizes such as; ResizePixel, Compressor.io, optimizilla, etc... -
Lazy Loading:
It's best practice to load images only when they enter the view port, you could use the html loading='lazy' attribute to achieve this.
<img src="img.png" loading="lazy"/>
-
Responsive Images:
Use responsive images to fit different screen sizes , you can use the media queries in CSS to achieve this.
/* Create a flex container */ .image-container { display: flex; justify-content: center; } /* Make the image responsive */ .image-container img { width: 100%; height: auto; max-width: 800px; } /* Make the image smaller on smaller screens */ @media (max-width: 600px) { .image-container img { max-width: 300px; } }
The above CSS makes the image responsive, centers it within the container, and adjusts its size based on the screen width to achieve responsiveness.
Alternatively, you can use the HTML "sizes" attribute:
<img src="image.jpg" srcset="image-small.jpg 400w, image-medium.jpg 800w, image-large.jpg 1200w" sizes="(max-width: 600px) 400px, (max-width: 900px) 800px, 1200px" alt="Responsive image">
The "srcset" attribute defines a set of image files and their sizes to be used depending on the viewport size, while the "sizes" attribute specifies the expected display size of the image for different viewport widths (in this case, 400px, 800px, 1200px).
This setup allows the browser to choose the most appropriate image based on the viewport size and resolution, optimizing both image quality and loading time.
2. Reduce Server Response Time
Server response time has a significant impact on the time it takes to load a page, here's how you can optimize it:
-
Upgrade your hosting plans:
:
It is important to upgrade your hosting plans to meet your websites needs. -
Adjust Server Configurations
:
Server settings such as max_connections, timeout and buffer sizes based on your needs. -
Monitor the performance
:
Use tools like Google PageSpeed Insights, GTmetrix, etc.... to monitor performance and provide insights and recommendations for your page.
3. Minify your code files
Your code files could harbor unnecessary characters, white spaces and redundant code which could increase their file size. There are various ways to fix this issue such as:
- Using Minification Tools:
Tools such as UglifyJS (for JavaScript), CSSNano (for CSS), HTMLMinifier (for HTML) help to automatically minify your files. - Using IDE Extensions:
Some IDE's (Integrated Development Environment) such as Visual Studio Code; have plugins or extensions that can minify code directly within the editor.
4. Use Asynchronous Loading for JavaScript files
This is good practice as it helps to load the page as JavaScript files are being fetched. Asynchronous loading can be achieved by using the:
- Async attribute
<script async src="script.js"></script>
- Defer attribute
<script defer src="script.js"></script>
Use defer for scripts that modify the DOM to ensure that the script is executed after the HTML document has finished parsing.
Avoid using async for scripts that modify the DOM, as it can cause unexpected behavior.
5. Enable Browser Caching
Caching allows browsers to store copies of files, reducing the need to download them again on subsequent visits.
You can implement by:
- Set Cache-Control Headers:
Define how long browsers should cache specific files. - Use a CDN:
Content Delivery Networks (CDNs) can serve cached content from locations closer to the user.
Apache Servers are the most commonly used web servers, here's how to programmatically implement browser caching in them:
- Create a .htaccess file in the root folder of your website.
-
Add the following code to set cache expiration times for various file types:
<IfModule mod_expires.c> ExpiresActive On ExpiresDefault "access plus 1 month" # Cache images ExpiresByType image/jpeg "access plus 1 year" ExpiresByType image/png "access plus 1 year" ExpiresByType image/gif "access plus 1 year" # Cache CSS and JavaScript ExpiresByType text/css "access plus 1 month" ExpiresByType application/javascript "access plus 1 month" </IfModule>
This configuration helps improve site performance by specifying how long different types of files should be cached by the browser.
In summary, employing these various strategies will definitely improve the overall speed and performance of your website.